LeetCode 482 License Key Formatting Solution in Python 3.
LeetCode 482 Solution 32 ms in Python 3
You are given a license key represented by the string s that contains only alphanumeric characters and dashes. You are also given an integer k.
Reformat the string s so that each group contains exactly k characters, except the first group that should be smaller than k but must contain at least 1 character.
Groups must be separated by dashes and all characters must be uppercase.
Analysis
First clean-up the input string s
- Remove all dashes from s,
- Convert s to uppercase.
#cleanup the input
chars = s.upper().replace('-','')
charslen = len(chars)
Find how many whole sets of k can be found in s.
#sets and remainder
pieces = charslen // k
rest = charslen % k
Using Modulo we find the remainder after dividing the lenght of s by k.
For the first set, insert the remainder characters lenght and a dash if there are more characters left.
#start with remainder
output = chars[:rest]
output+= '-' if rest != 0 else ''
Slice the remaining string using a loop thru the sets. i*k is to jump from set to set and +rest is to offset the already used characters.
#slice the remaining string into sets
for i in range(pieces):
output += chars[i*k+rest : (i+1)*k+rest] + '-'
output = output[:-1] # remove the last - char
Whole Solution Code in Python 3
Code steps
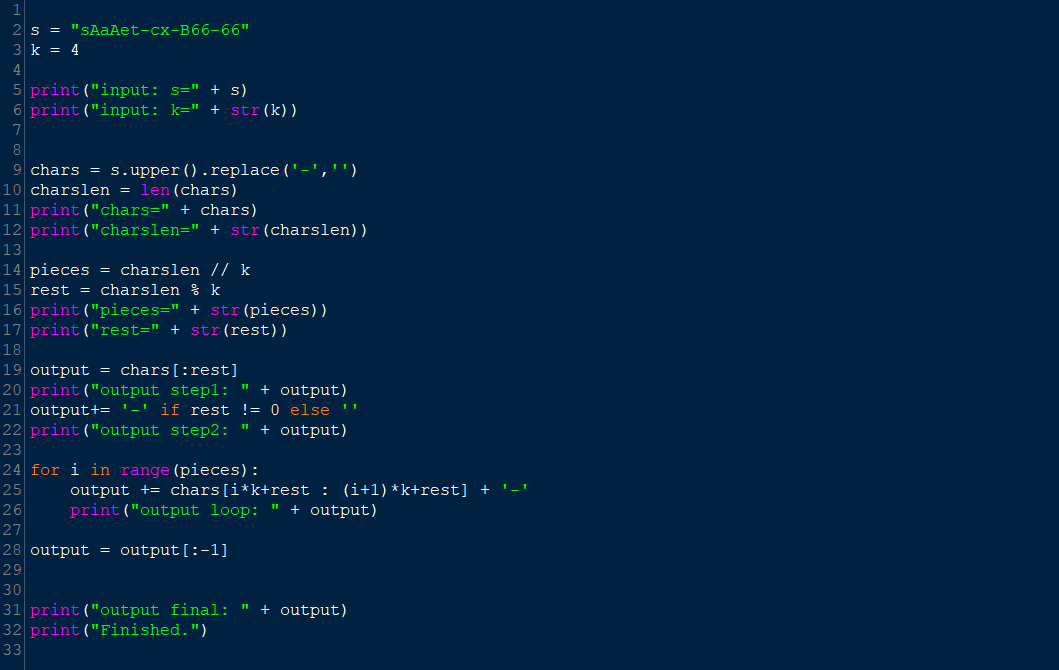
Output
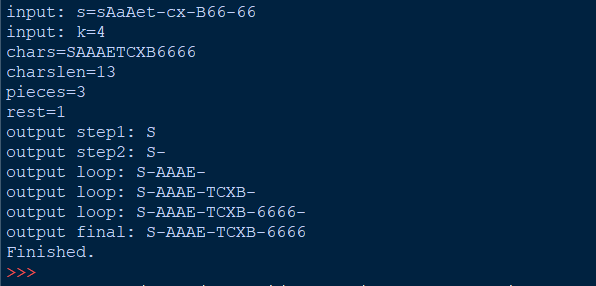
class Solution:
def licenseKeyFormatting(self, s: str, k: int) -> str:
"""
Dragos Ion 2021
32 ms solution
Using Mod and Slice
"""
#cleanup the input
chars = s.upper().replace('-','')
charslen = len(chars)
#sets and remainder
pieces = charslen // k
rest = charslen % k
#start with remainder
output = chars[:rest]
output+= '-' if rest != 0 else ''
#slice the remaining string into sets
for i in range(pieces):
output += chars[i*k+rest : (i+1)*k+rest] + '-'
output = output[:-1] # remove the last - char
return output
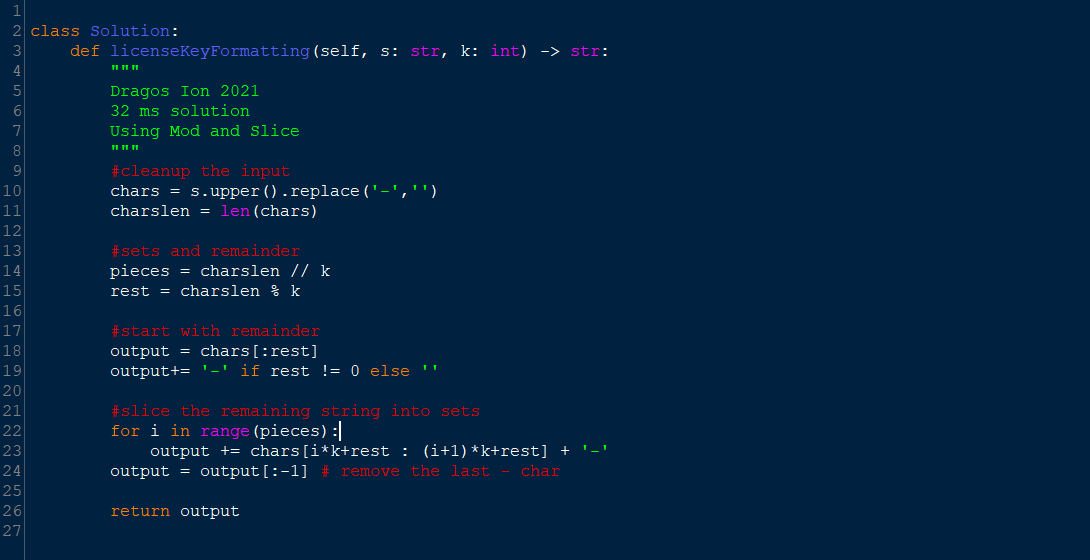
Leave a Reply